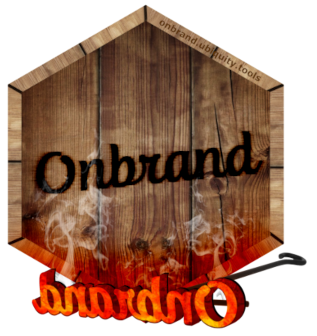
Add Content to Body of a Word Document Report
Source:R/report_add_doc_content.R
report_add_doc_content.Rd
Appends content to the body of a Word document
Usage
report_add_doc_content(
obnd,
type = NULL,
content = NULL,
fig_start_at = NULL,
tab_start_at = NULL,
verbose = TRUE
)
Arguments
- obnd
onbrand report object
- type
Type of content to add
- content
Content to add
- fig_start_at
Indicates that you want to restart figure numbering at the specified value (e.g. 1) after adding this content a value of
NULL
(default) will ignore this option.- tab_start_at
Indicates that you want to restart figure numbering at the specified value (e.g. 1) after adding this content a value of
NULL
(default) will ignore this option.- verbose
Boolean variable when set to TRUE (default) messages will be displayed on the terminal; Messages will be included in the returned onbrand object.
Value
onbrand object with the content added to the body or isgood set to FALSE with any messages in the msgs field. The isgood value is a Boolean variable indicating the current state of the object.
Details
For each content
types listed below the different content
outlined is expected. Text
can be specified in different formats: "text"
indicates plain text,
"fpar"
is formatted text defined by the fpar
command from the
officer
package, "ftext"
is a list of formatted text defined
by the ftext
command, and "md"
is text formatted in markdown
format (?md_to_officer
for markdown details).
"break"
page break, content is (NULL
) and a page break will be inserted here"ph"
adds placeholder text substitution"name"
placeholder name (value in body of text surrounded by three equal signs, e.g. if you have"===MYPH==="
. in the document the name is just"MYPH"
)"value"
value to be substituted into the placeholder ("my text"
)"location"
document location where the placeholder will be located (either"header"
,"footer"
, or"body"
)
"toc"
generates the table of contents, and content is a list that must contain __one__ of the following."level"
number indicating the depth of the contents to display (default:3
)"style"
string containing the onbrand style name to use to build the TOC
"section"
formats the current document section"section_type"
type of section to apply, either"columns"
,"continuous"
,"landscape"
,"portrait"
,"columns"
, or"columns_landscape"
"width"
override the default page width with this value in inches (NULL
)"height"
override the default page height with this value in inches (NULL
)"widths"
column widths in inches, number of columns set by number of values (NULL
)"space"
space in inches between columns (NULL
)"sep"
Boolean value controlling line separating columns (FALSE
)
"text"
content is a list containing a paragraph of text with the following elements"text"
string containing the text content either a string or the output of"fpar"
for formatted text."style"
string containing the style to use (defaultNULL
will use thedoc_def
,Text
style)"format"
string containing the format, either"text"
,"fpar"
, or"md"
(defaultNULL
assumes"text"
format)
"imagefile"
content is a list containing information describing an image file with the following elementsimage
string containing path to image filecaption
caption of the image (NULL
)caption_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)notes
notes to add under the image (NULL
)notes_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)key
unique key for cross referencing e.g. "FIG_DATA" (NULL
)height
height of the image (NULL
)width
width of the image (NULL
)
"ggplot"
content is a list containing a ggplot object, (eg. p = ggplot() + ....) with the following elementsimage
ggplot objectcaption
caption of the image (NULL
)caption_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)notes
notes to add under the image (NULL
)notes_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)key
unique key for cross referencing e.g. "FIG_DATA" (NULL
)height
height of the image (NULL
)width
width of the image (NULL
)
"table"
content is a list containing the table content and other options with the following elements:table
data frame containing the tabular data"style"
string containing the style to use (defaultNULL
will use thedoc_def
,Table
style)caption
caption of the table (NULL
)caption_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)notes
notes to add under the image (NULL
)notes_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)key
unique key for cross referencing e.g. "TAB_DATA" (NULL
)header
Boolean variable to control displaying the header (TRUE
)first_row
Boolean variable to indicate that the first row contains header information (TRUE
)
"flextable"
content is a list containing flextable content and other options with the following elements (defaults in parenthesis):table
data frame containing the tabular datacaption
caption of the table (NULL
)caption_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)notes
notes to add under the image (NULL
)notes_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)key
unique key for cross referencing e.g. "TAB_DATA" (NULL
)header_top
,header_middle
,header_bottom
(NULL
) a list with the same names as the data frame names containing the tabular data and values with the header text to show in the tableheader_format
string containing the format, either"text"
, or"md"
(defaultNULL
assumes"text"
format)merge_header
(TRUE
) Set to true to combine column headers with the same informationtable_body_alignment
, table_header_alignment ("center") Controls alignmenttable_autofit
(TRUE
) Automatically fit content, or specify the cell width and height withcwidth
(0.75
) andcheight
(0.25
)table_theme
("theme_vanilla"
) Table theme
"flextable_object"
content is a list specifying the a user defined flextable object with the following elements:ft
flextable objectcaption
caption of the table (NULL
)caption_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)notes
notes to add under the image (NULL
)notes_format
string containing the format, either"text"
,"ftext"
, or"md"
(defaultNULL
assumes"text"
format)key
unique key for cross referencing e.g. "TAB_DATA" (NULL
)
Examples
# Read Word template into an onbrand object
obnd = read_template(
template = file.path(system.file(package="onbrand"), "templates", "report.docx"),
mapping = file.path(system.file(package="onbrand"), "templates", "report.yaml"))
# The examples below use the following packages
library(ggplot2)
library(flextable)
library(officer)
# Adding text
obnd = report_add_doc_content(obnd,
type = "text",
content = list(text="Text with no style specified will use the doc_def text format."))
# Text formatted with fpar
fpartext = fpar(
ftext("Formatted text can be created using the ", prop=NULL),
ftext("fpar ", prop=fp_text(color="green")),
ftext("command from the officer package.", prop=NULL))
obnd = report_add_doc_content(obnd,
type = "text",
content = list(text = fpartext,
format = "fpar",
style = "Normal"))
# Text formatted with markdown
mdtext = "Formatted text can be created using
**<color:green>markdown</color>** formatting"
obnd = report_add_doc_content(obnd,
type = "text",
content = list(text = mdtext,
format = "md",
style = "Normal"))
# Adding figures
p = ggplot() + annotate("text", x=0, y=0, label = "picture example")
imgfile = tempfile(pattern="image", fileext=".png")
ggsave(filename=imgfile, plot=p, height=5.15, width=9, units="in")
# From an image file:
obnd = report_add_doc_content(obnd,
type = "imagefile",
content = list(image = imgfile,
caption = "This is an example of an image from a file."))
# From a ggplot object
obnd = report_add_doc_content(obnd,
type = "imagefile",
content = list(image = imgfile,
caption = "This is an example of an image from a file."))
#Adding tables
tdf = data.frame(Parameters = c("Length", "Width", "Height"),
Values = 1:3,
Units = c("m", "m", "m") )
# Word table
tab_cont = list(table = tdf,
caption = "Word Table.")
obnd = report_add_doc_content(obnd,
type = "table",
content = tab_cont)
# onbrand flextable abstraction:
tab_cont = list(table = tdf,
caption = "Word Table.")
obnd = report_add_doc_content(obnd,
type = "table",
content = tab_cont)
# flextable object
tab_fto = flextable(tdf)
obnd = report_add_doc_content(obnd,
type = "flextable_object",
content = list(ft=tab_fto,
caption = "Flextable object created by the user."))
# Saving the report output
save_report(obnd, tempfile(fileext = ".docx"))
#> $isgood
#> [1] TRUE
#>
#> $msgs
#> NULL
#>